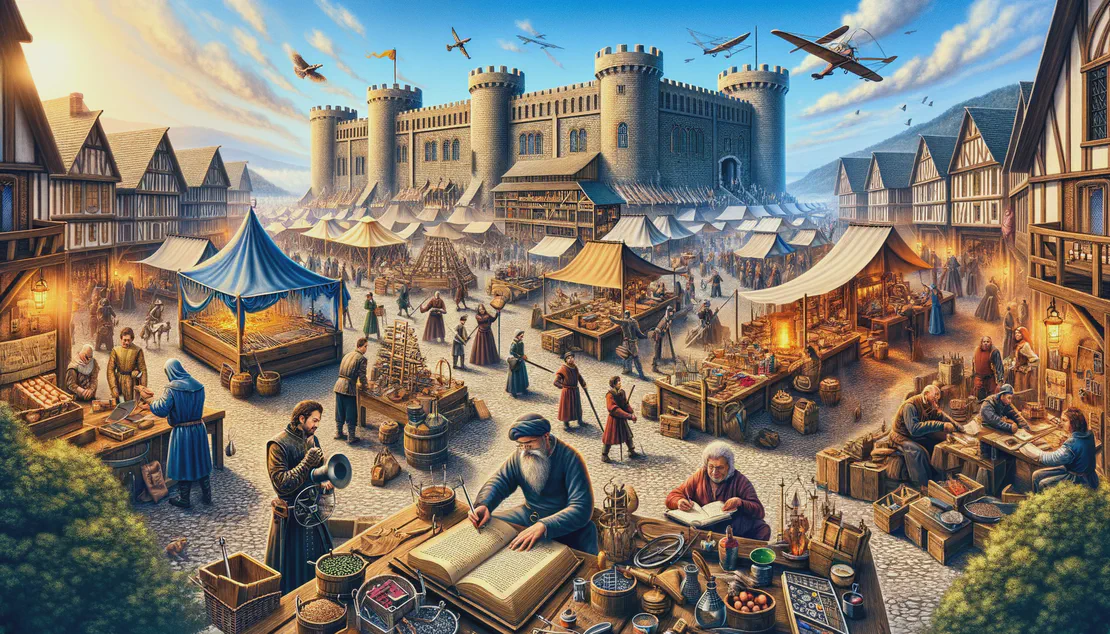
Compelling Use Cases Where Golang's Concurrency Features Truly Shine
- Serjo Agronov
- Go , Back end , Web frameworks
- February 25, 2024
- Reading time: 4 minutes
Part of the series: Go Concurrency
- Part 1: Concurrent Programming in Go
- Part 2: This Article
Table of Contents
Understanding Golang Concurrency
At its heart, concurrency signifies the ability for a program to handle multiple tasks seemingly “at the same time.” This differs from true parallelism (multiple tasks executing simultaneously across multiple CPU cores). Golang’s strength lies in its lightweight approach to concurrency:
- Goroutines: Think of goroutines as extremely cheap ’threads.’ Spawning thousands, even tens of thousands, of goroutines incurs minimal overhead compared to traditional operating system threads.
- Channels: Channels are the communication conduits between goroutines. They provide a safe mechanism for sending and receiving data, enabling elegant coordination and synchronization.
Key Use Cases for Golang Concurrency
Network-Bound Applications
Golang excels in network programming, where tasks often involve waiting for I/O (disk or network ) operations:
- High-Performance Web Servers: Goroutines can efficiently handle numerous simultaneous client connections. Incoming requests are mapped to goroutines, which process them swiftly, minimizing the impact of slow clients on overall server responsiveness.
- APIs and Microservices: Golang’s concurrency constructs are perfect for building scalable APIs and microservices. Individual requests are handled by lightweight goroutines, allowing your systems to gracefully serve a large number of users.
- Real-Time Communication: WebSockets protocols (used for chats, dashboards, etc.) are easily managed with Golang. Each WebSocket connection can be represented by a goroutine, facilitating broadcasting messages or handling individual client interactions.
Parallel Processing Tasks
While Golang can leverage multiple CPU cores, its concurrency model shines for tasks that can be broken into smaller, independent sub-tasks:
- Data Processing and Pipelines: Process large datasets by splitting work among multiple goroutines. Goroutines can pull data from files or databases, perform transformations, and pass results through channels for aggregation or further processing.
- Image and Video Processing: Divide the processing of a large image or video file into smaller chunks, with goroutines working on each chunk independently. This can significantly reduce processing time.
- Scientific Simulations and Computations: Simulations often involve numerous calculations that can be executed concurrently. Goroutines are a natural fit for modeling and distributing these computations.
Task Scheduling and Coordination
Manage complex workflows with Golang’s concurrency primitives:
- Background Jobs and Task Queues: Goroutines are perfect for background workers pulling tasks from a queue. This decouples task execution from incoming requests, improving responsiveness.
- Batch Processing: Schedule batches of work to run at specific times or with regular intervals using Golang’s ’time’ package and goroutines.
- Resource Throttling: Employ channels in conjunction with goroutines to limit the number of concurrent requests to external APIs or resources, preventing unintentional overload.
Asynchronous Operations and Event Handling
Enhance user experience and system efficiency with asynchronous programming:
- Non-Blocking Web Requests: Perform multiple external web requests concurrently (e.g., fetching data from different APIs). This prevents your application from freezing while waiting for responses.
- Timeout Handling: Use goroutines with channels and timers to manage timeouts gracefully, avoiding locked-up resources.
- Event-Driven Architectures: Model systems reacting to events (user input, sensor data) using goroutines and channels to coordinate responses.
System-Level Programming and Tooling
Golang’s concurrency lends itself well to tasks close to the system level:
- Network Utilities and Proxies: Efficiently handle multiple network connections in network monitoring tools, proxies, and load balancers with scalable goroutine-based design.
- System Monitoring and Automation: Create lightweight monitoring agents that concurrently check system resources or schedule automation tasks using goroutines.
- Command-Line Tools (CLIs): Develop responsive CLIs performing background operations concurrently with the main user interaction loop.
Important Considerations
- Synchronization: While channels make coordination much safer, always be mindful of race conditions and deadlocks when working with shared data.
- Not a Magic Bullet: Concurrency introduces complexity. Assess whether it’s truly necessary before diving in. Simple, sequential code is often easier to reason about.
- CPU-Bound Workloads: For purely computation-intensive tasks, exploring true parallelism across cores might be more beneficial.
Conclusion
Golang’s elegant concurrency model empowers you to build highly efficient and scalable systems. From web servers and microservices to data processing and system-level tools, Golang shines when you need to juggle multiple tasks or leverage asynchronous patterns. By understanding these key use cases, you unleash the power of lightweight concurrency to create truly responsive and performant applications.