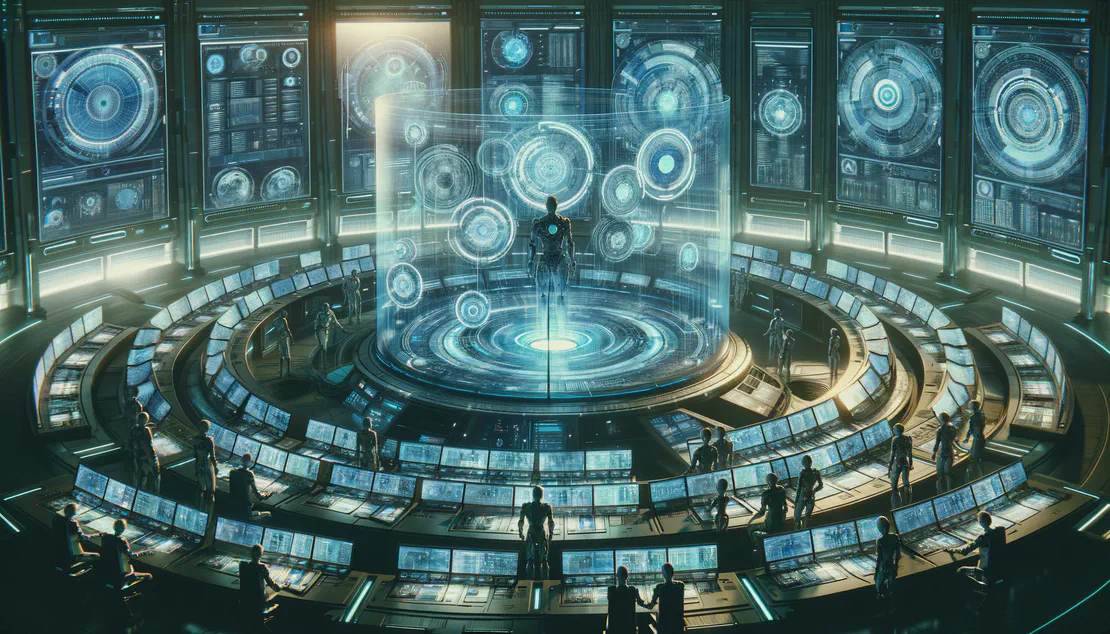
Concurrent Programming in Go
- Serjo Agronov
- Go , Concurrency , Back end
- February 28, 2024
- Reading time: 6 minutes
Part of the series: Go Concurrency
- Part 1: This Article
- Part 2: Compelling Use Cases Where Golang's Concurrency Features Truly Shine
Table of Contents
Mastering Concurrency in Go: A Comprehensive Guide
Concurrency is a core principle in software engineering, enabling applications to perform multiple tasks simultaneously, improving throughput and efficiency, particularly in IO-bound and high-latency operations. Go, with its built-in support for concurrency, offers a robust set of features designed to make concurrent programming more accessible and safe. This guide will take you from the basics of Go concurrency to advanced patterns, providing you with the knowledge you need to master concurrent programming in Go.
Introduction to Concurrency in Go
At the heart of Go’s concurrency model is the goroutine, a lightweight thread managed by the Go runtime. Goroutines allow you to perform tasks concurrently, with a much lower overhead than traditional OS threads. This efficiency enables the creation of applications that can handle thousands of concurrent operations.
Understanding Goroutines
A goroutine is started with the go
keyword followed by a function call. This simplicity masks the power of goroutines, enabling developers to create concurrent applications with ease.
package main
import (
"fmt"
"time"
)
func say(s string) {
for i := 0; i < 5; i++ {
time.Sleep(100 * time.Millisecond)
fmt.Println(s)
}
}
func main() {
go say("world")
say("hello")
}
In this example, say("world")
runs concurrently withsay("hello")
, demonstrating the basic use of goroutines.
Channels: Communication Between Goroutines
Channels are Go’s way of allowing goroutines to communicate with each other, enabling the synchronization of operations. A channel is created using the chan
keyword, and its type specifies the type of values that can be passed through it.
package main
import "fmt"
func sum(s []int, c chan int) {
sum := 0
for _, v := range s {
sum += v
}
c <- sum // send sum to c
}
func main() {
s := []int{7, 2, 8, -9, 4, 0}
c := make(chan int)
go sum(s[:len(s)/2], c)
go sum(s[len(s)/2:], c)
x, y := <-c, <-c // receive from c
fmt.Println(x, y, x+y)
}
This example demonstrates using channels to synchronize data computation across multiple goroutines.
Advanced Concurrency Patterns in Go
Select Statement
The select
statement in Go allows a goroutine to wait on multiple communication operations, akin to a switch statement but for channels.
package main
import (
"fmt"
"time"
)
func main() {
c1 := make(chan string)
c2 := make(chan string)
go func() {
time.Sleep(1 * time.Second)
c1 <- "one"
}()
go func() {
time.Sleep(2 * time.Second)
c2 <- "two"
}()
for i := 0; i < 2; i++ {
select {
case msg1 := <-c1:
fmt.Println("Received", msg1)
case msg2 := <-c2:
fmt.Println("Received", msg2)
}
}
}
Buffering and Deadlock
Understanding channel buffering and avoiding deadlocks are crucial in building reliable concurrent applications. A buffered channel has a capacity, allowing values to be sent without immediate receipt until the buffer is full.
Deadlocks occur when goroutines wait on each other to send or receive operations, leading to a standstill. Recognizing and avoiding these scenarios is essential for robust application design.
Advanced Synchronization Techniques
Beyond basic channels, Go provides advanced synchronization techniques through the sync package, including mutexes and wait groups, for fine-grained control over goroutine behavior and resource access.
Mutexes and wait groups
Mutexes and wait groups are crucial synchronization primitives in Go, enabling safe access to shared resources among goroutines and managing goroutine lifecycles, respectively. Let’s explore two use cases that demonstrate how to use mutexes and wait groups effectively in Go programming.
Safe Access to a Shared Resource with Mutex
Scenario: Imagine you’re building a counter service where multiple goroutines increment a shared counter. To prevent race conditions, you can use a mutex to ensure that only one goroutine can access the counter at a time.
package main
import (
"fmt"
"sync"
)
// SafeCounter is safe to use concurrently.
type SafeCounter struct {
v map[string]int
mux sync.Mutex
}
// Inc increments the counter for the given key.
func (c *SafeCounter) Inc(key string) {
c.mux.Lock()
// Lock so only one goroutine at a time can access the map c.v.
c.v[key]++
c.mux.Unlock()
}
// Value returns the current value of the counter for the given key.
func (c *SafeCounter) Value(key string) int {
c.mux.Lock()
// Lock so only one goroutine at a time can access the map c.v.
defer c.mux.Unlock()
return c.v[key]
}
func main() {
c := SafeCounter{v: make(map[string]int)}
for i := 0; i < 1000; i++ {
go c.Inc("somekey")
}
// Wait for a second to ensure all goroutines have finished
time.Sleep(time.Second)
fmt.Println(c.Value("somekey"))
}
In this example, SafeCounter
uses a sync.Mutex
to synchronize access to its v
map, ensuring that concurrent map updates do not lead to race conditions.
Coordinating Task Completion with WaitGroup
Scenario: You’re tasked with processing multiple batches of data concurrently. You need to wait for all batches to be processed before aggregating the results. A sync.WaitGroup
is perfect for waiting for a collection of goroutines to finish executing.
package main
import (
"fmt"
"sync"
"time"
)
// processData simulates processing data and takes a duration as an argument.
func processData(i int, wg *sync.WaitGroup) {
defer wg.Done() // Notify the WaitGroup that the goroutine has finished executing.
fmt.Printf("Processing batch %d\n", i)
// Simulate a time-consuming task
time.Sleep(time.Duration(i) * time.Millisecond * 100)
fmt.Printf("Batch %d processed\n", i)
}
func main() {
var wg sync.WaitGroup
for i := 1; i <= 5; i++ {
wg.Add(1) // Increment the WaitGroup counter.
go processData(i, &wg)
}
wg.Wait() // Block until the WaitGroup counter returns to 0; all goroutines have finished.
fmt.Println("All batches processed.")
}
This example demonstrates how sync.WaitGroup
is used to wait for all data processing goroutines to complete before continuing execution, ensuring that the final aggregation only happens after all processing is done.
Both use cases illustrate the importance of mutexes and wait groups in concurrent programming, providing mechanisms to safely access shared resources and coordinate the execution flow of goroutines.
Best Practices for Concurrency in Go
- Start Small: Begin with a simple model of your concurrent operations and gradually add complexity.
- Avoid Sharing State: Design your goroutines to avoid the need for shared state when possible, reducing the risk of race conditions.
- Make Use of
select
: Utilize theselect
statement for more readable and efficient concurrent logic. - Properly Handle Goroutine Lifecycles: Ensure goroutines complete appropriately to avoid memory leaks or unintended resource consumption.
- Profile and Test: Regularly profile and test your concurrent Go applications to identify bottlenecks, race conditions, and other issues.
Conclusion
Concurrency in Go is a powerful feature that, when used correctly, can greatly enhance the performance and responsiveness of applications. By understanding the basic and advanced concepts outlined in this guide, you’re now equipped to take full advantage of Go’s concurrency model in your projects. Remember, the key to mastering concurrency is practice and continuous learning, so don’t hesitate to experiment with these concepts in your Go applications.